Image Card Component
Display images with optional title, description, and action buttons
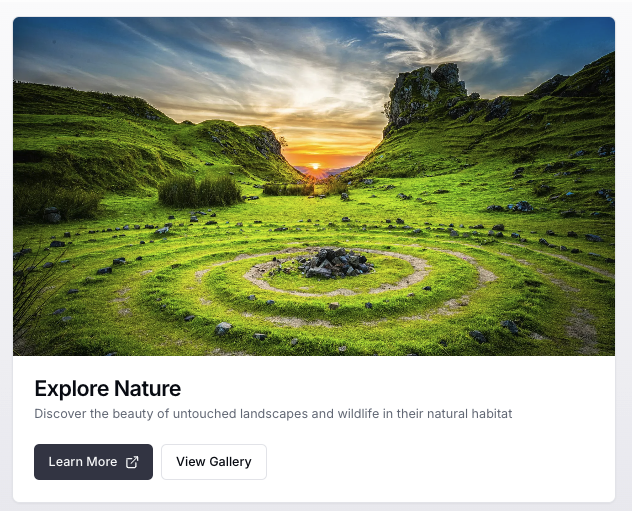
Overview
The Image Card component displays images with configurable aspect ratios, optional overlay text, and action buttons. It supports hover effects and multiple layout options for presenting visual content.
Usage
To use the Image Card component in your tool handler, follow these steps:
- Import the
ImageCardUIBuilder
andDainResponse
from the@dainprotocol/utils
package:
import { ImageCardUIBuilder, DainResponse } from "@dainprotocol/utils";
- Create an ImageCardUIBuilder instance and configure it:
const imageCardUI = new ImageCardUIBuilder({ imageUrl: "https://example.com/image.jpg"}) // Mandatory: image URL
.title("Mountain Landscape") // Optional
.description("Beautiful vista") // Optional
.imageAlt("Mountain sunset") // Optional
.aspectRatio("video") // Optional: square | video | wide | portrait
.withOverlay(true) // Optional
.build();
- Return the ImageCardUIBuilder instance in a DainResponse object:
return new DainResponse({
text: "Generated image card",
data: { /* Your data */ },
ui: imageCardUI
});
Configuration
Mandatory Props
- imageUrl (string): URL of the image to display
Optional Props
- title (string): Card title
- description (string): Card description
- imageAlt (string): Alt text for the image
- aspectRatio (
square
|video
|wide
|portrait
): Image aspect ratio - overlay (boolean): Whether to show text overlay on image
- className (string): Custom CSS class name
Aspect Ratios
square
: 1:1video
: 16:9wide
: 21:9portrait
: 3:4
Actions
The Image Card supports two types of actions:
imageCardUI.onLearnMore({
tool: "yourToolName",
params: {
// Predefined parameters
id: "image123"
}
});
imageCardUI.onViewGallery({
tool: "galleryViewer",
params: {
// Predefined parameters
galleryId: "nature"
}
});
Action Arguments
- tool (string): The name of the tool to be called when the action is triggered
- params (object): Pre-defined parameters that will be included with the action:
- These values are set at build time
- Will be passed to the tool when the action is triggered
- paramSchema (object): Optional schema defining required parameters
Examples
Basic Image Card
const basicCard = new ImageCardUIBuilder("https://example.com/photo.jpg")
.title("Nature Photo")
.description("Captured in the wild")
.imageAlt("Wildlife photograph")
.build();
return new DainResponse({
text: "Basic Image Card",
data: {},
ui: basicCard
});
Image Card with Overlay and Action
const overlayCard = new ImageCardUIBuilder("https://example.com/landscape.jpg")
.title("Scenic Vista")
.description("Breathtaking mountain views")
.aspectRatio("wide")
.withOverlay(true)
.onLearnMore({
tool: "contentViewer",
params: {
type: "landscape",
id: "vista123"
}
})
.build();
return new DainResponse({
text: "Interactive Image Card",
data: {},
ui: overlayCard
});
Gallery Image Card
const galleryCard = new ImageCardUIBuilder("https://example.com/gallery-preview.jpg")
.title("Photo Collection")
.description("View all photos in this collection")
.aspectRatio("square")
.onViewGallery({
tool: "galleryViewer",
params: {
galleryId: "nature2023",
source: "featured"
}
})
.build();
return new DainResponse({
text: "Gallery Preview Card",
data: {},
ui: galleryCard
});