Timeline Component
Display chronological events with timestamps, titles, and descriptions
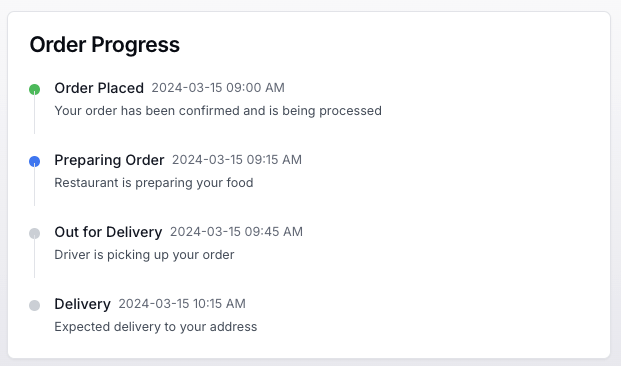
Overview
The Timeline component displays a vertical list of chronological events, each with a timestamp, optional title, and description. It's perfect for showing history, activity logs, or step-by-step processes.
Usage
To use the Timeline component in your tool handler, follow these steps:
- Import the
TimeLineUIBuilder
andDainResponse
from the@dainprotocol/utils
package:
import { TimeLineUIBuilder, DainResponse } from "@dainprotocol/utils";
- Create a TimeLineUIBuilder instance and configure it:
const timelineUI = new TimeLineUIBuilder()
.title("Project History") // Optional
.description("Key milestones") // Optional
.addItems([ // Mandatory
{
timestamp: "2024-03-01",
title: "Project Started",
description: "Initial planning phase began"
},
{
timestamp: "2024-03-15",
title: "Phase 1 Complete",
description: "Core features implemented"
}
])
.build();
- Return the TimeLineUIBuilder instance in a DainResponse object:
return new DainResponse({
text: "Generated timeline",
data: { /* Your data */ },
ui: timelineUI
});
Configuration
Mandatory Props
- items (TimeLineItem[]): Array of timeline items
Optional Props
- title (string): Timeline title
- description (string): Timeline description
TimeLineItem Interface
Each timeline item is defined using this interface:
interface TimeLineItem {
timestamp: string; // Mandatory: date/time of the event
title?: string; // Optional: event title
description?: string; // Optional: event description
}
Actions
The Timeline supports item click actions:
timelineUI.onItemClick({
tool: "eventViewer",
params: {
// Predefined parameters
view: "detail"
},
paramSchema: {
view: { type: "string" }
}
});
Action Arguments
- tool (string): The name of the tool to be called when an item is clicked
- params (object): Pre-defined parameters that will be included with the action:
- These values are set at build time
- Will be passed to the tool when an item is clicked
- paramSchema (object): Schema defining required parameters
Examples
Basic Timeline
const basicTimeline = new TimeLineUIBuilder()
.addItems([
{
timestamp: "2024-01-01",
title: "New Year Launch",
description: "Product officially launched"
},
{
timestamp: "2024-02-15",
title: "First Update",
description: "Major features added"
}
])
.build();
return new DainResponse({
text: "Basic Timeline",
data: {},
ui: basicTimeline
});
Interactive Timeline
const interactiveTimeline = new TimeLineUIBuilder()
.title("User Activity")
.description("Recent account activities")
.addItems([
{
timestamp: "2024-03-10T14:30:00",
title: "Login",
description: "User logged in from new device"
},
{
timestamp: "2024-03-10T15:45:00",
title: "Settings Changed",
description: "Profile settings updated"
}
])
.onItemClick({
tool: "activityViewer",
params: {
type: "user_activity"
}
})
.build();
return new DainResponse({
text: "Activity Timeline",
data: {},
ui: interactiveTimeline
});
Project Milestones
const milestoneTimeline = new TimeLineUIBuilder()
.title("Project Roadmap")
.description("Development milestones and deadlines")
.addItems([
{
timestamp: "2024-Q1",
title: "Planning Phase",
description: "Requirements gathering and architecture design"
},
{
timestamp: "2024-Q2",
title: "Development",
description: "Core features implementation"
},
{
timestamp: "2024-Q3",
title: "Testing",
description: "QA and user acceptance testing"
}
])
.onItemClick({
tool: "projectViewer",
params: {
view: "milestone"
}
})
.build();
return new DainResponse({
text: "Project Timeline",
data: {},
ui: milestoneTimeline
});